Compiling ffmpeg with NVIDIA GPU Hardware Acceleration on Ubuntu 20.04LTS
Please note that the following commands were executed on a system that already had CUDA support so we might be missing a step or two to enable NVIDIA CUDA support.
Install necessary packages
sudo apt-get install build-essential yasm cmake libtool libc6 libc6-dev unzip wget libnuma1 libnuma-dev nvidia-cuda-toolkit;
Clone and install ffnvcodec
git clone https://git.videolan.org/git/ffmpeg/nv-codec-headers.git;
cd nv-codec-headers;
sudo make install;
cd -;
Clone and compile FFmpeg’s public GIT repository with NVIDIA GPU hardware acceleration
git clone https://git.ffmpeg.org/ffmpeg.git ffmpeg/;
cd ffmpeg;
./configure --enable-nonfree --enable-cuda-nvcc --enable-libnpp --extra-cflags=-I/usr/local/cuda/include --extra-ldflags=-L/usr/local/cuda/lib64 --disable-static --enable-shared;
make -j 8;
sudo make install;
SUCCESS!
After performing the above steps, we were able to process media using ffmpeg
without stressing our CPU! The workload was transferred to the GPU!
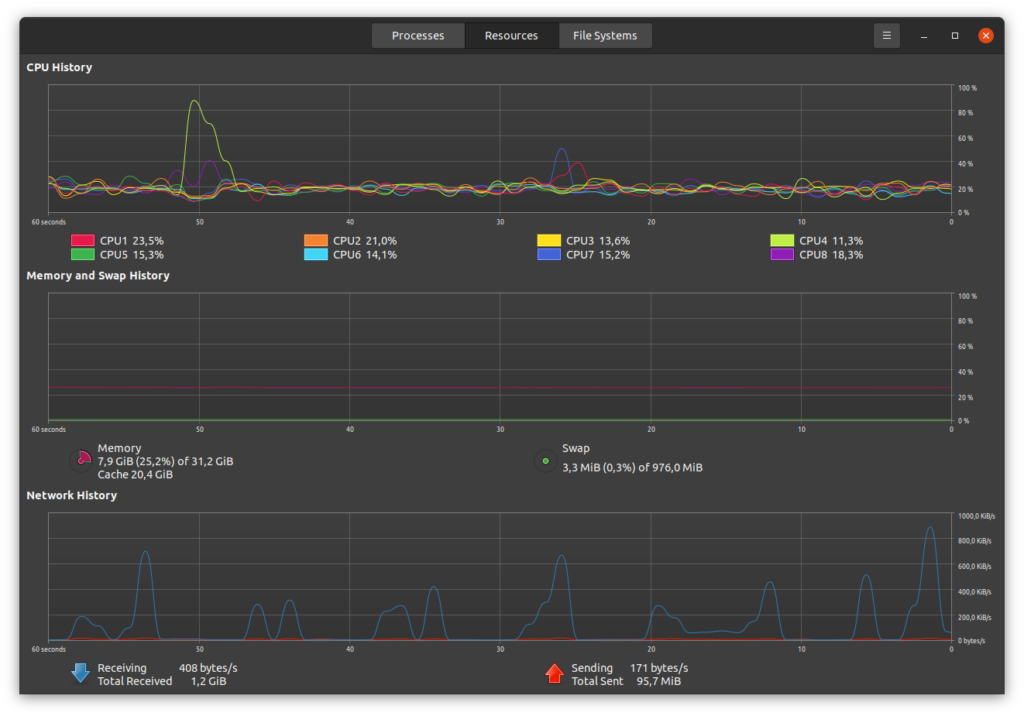