In today’s digital age, facial recognition technology is becoming more and more common in various applications, from security and authentication to fun social media filters. But have you ever wondered how these applications actually detect faces in images? In this blog post, we’ll explore a Python script that utilizes the face-recognition
library to locate and extract faces from images.
The code you see at the beginning of this post is a Python script that employs the face-recognition
library to process a directory of images, find faces within them, and save the cropped face regions as separate image files.
Prerequisites
Before we dive into the code, there are a few prerequisites you need to have in place:
- Python: You should have Python installed on your system.
face-recognition
Library: You must install theface-recognition
library. You can do this by running the following command:
pip install face-recognition;
Understanding the Code
Now, let’s break down the code step by step to understand what each part does:
#!/bin/python
from PIL import Image
import face_recognition
import sys
import os
inputDirectory = sys.argv[1]
outputDirectory = sys.argv[2]
- The code begins by importing necessary libraries like
PIL
(Pillow),face_recognition
,sys
, andos
. - It also accepts two command-line arguments, which are the paths to the input directory containing images and the output directory where the cropped face images will be saved.
for filename in os.listdir(inputDirectory):
path = os.path.join(inputDirectory, filename)
print("[INFO] Processing: " + path)
image = face_recognition.load_image_file(path)
faces = face_recognition.face_locations(image, model="cnn")
print("[INFO] Found {0} Faces.".format(len(faces)))
- The code then iterates through the files in the input directory using
os.listdir()
. For each file, it constructs the full path to the image. - It loads the image using
face_recognition.load_image_file(path)
. - The
face_recognition.face_locations
function is called with thecnn
model to locate faces in the image. Thecnn
model is more accurate than the default HOG-based model. - The number of detected faces is printed for each image.
for (top, right, bottom, left) in faces:
print("A face is located at pixel location Top: {}, Left: {}, Bottom: {}, Right: {}".format(top, left, bottom, right))
face_image = image[top:bottom, left:right]
pil_image = Image.fromarray(face_image)
pil_image.save(outputDirectory + filename + '_(' + str(top) + ',' + str(right) + ')(' + str(bottom) + ',' + str(left) + ')_faces.jpg')
- If faces are detected in the image, the code enters a loop to process each face.
- It prints the pixel locations of the detected face.
- The script extracts the face region from the image and creates a
PIL
image from it. - Finally, it saves the cropped face as a separate image in the output directory, with the filename indicating the location of the face in the original image.
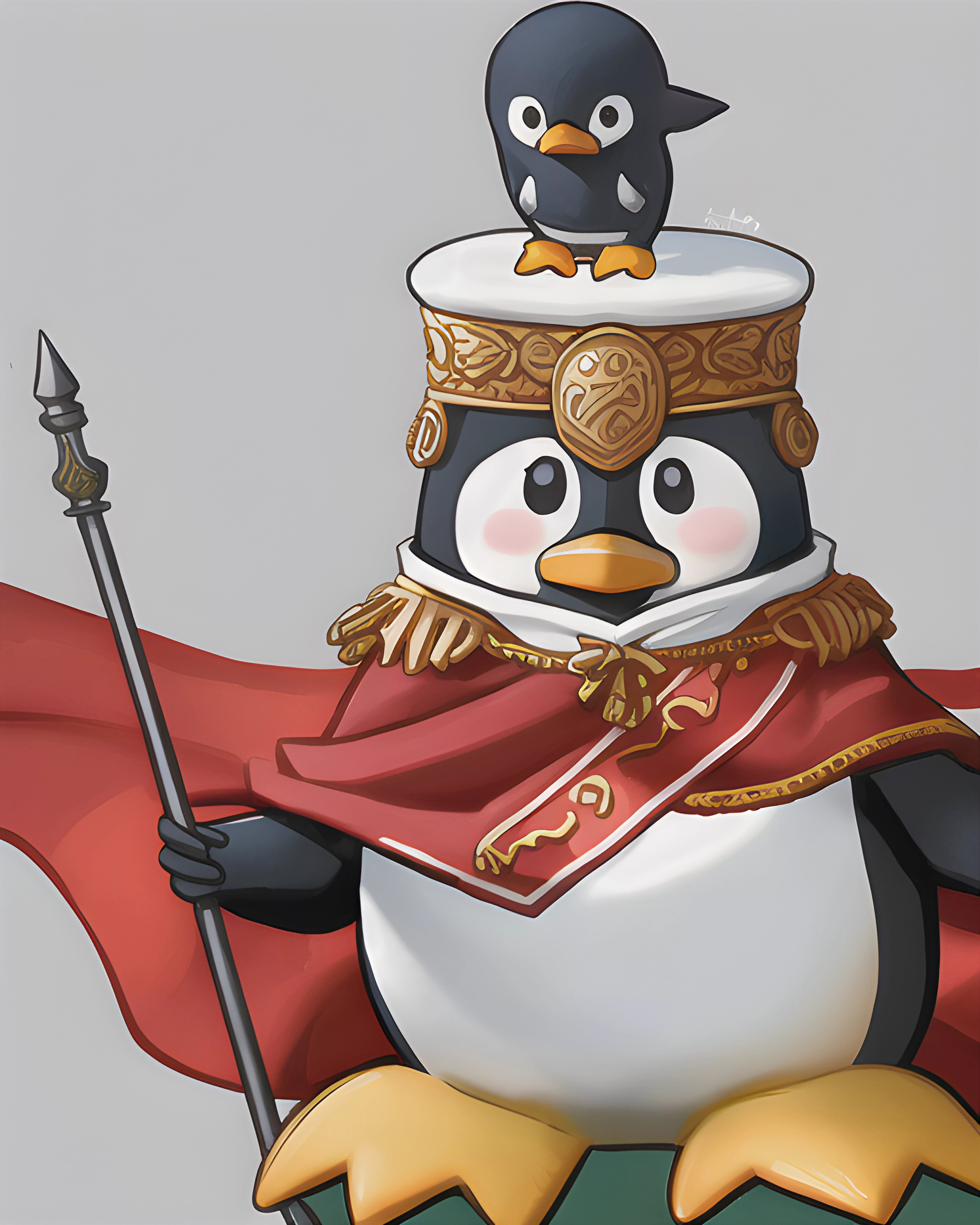
Running the Code
To run this script, you need to execute it from the command line, providing two arguments: the input directory containing images and the output directory where you want to save the cropped faces. Here’s an example of how you might run the script:
python face_extraction.py input_images/ output_faces/;
This will process all the images in the input_images
directory and save the cropped faces in the output_faces
directory.
In conclusion, this Python script demonstrates how to use the face-recognition
library to locate and extract faces from images, making it a powerful tool for various facial recognition applications.
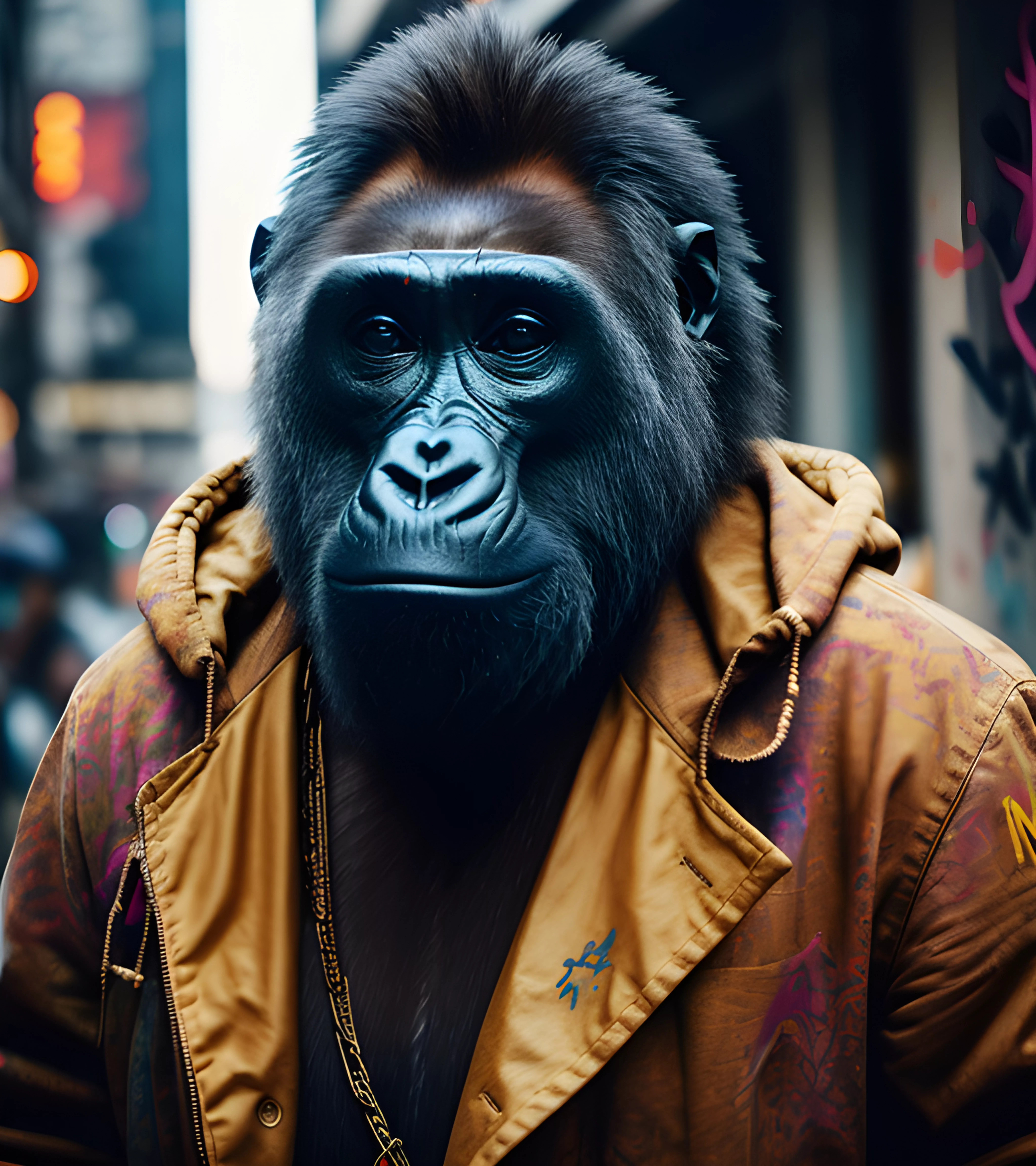
Full Code
#!/bin/python
# Need to install the following:
# pip install face-recognition
from PIL import Image
import face_recognition
import sys
import os
inputDirectory = sys.argv[1];
outputDirectory = sys.argv[2];
for filename in os.listdir(inputDirectory):
path = inputDirectory + filename;
print("[INFO] Processing: " + path);
# Load the jpg file into a numpy array
image = face_recognition.load_image_file(path)
# Find all the faces in the image using the default HOG-based model.
# This method is fairly accurate, but not as accurate as the CNN model and not GPU accelerated.
#faces = face_recognition.face_locations(image)
faces = face_recognition.face_locations(image, model="cnn")
print("[INFO] Found {0} Faces.".format(len(faces)));
for (top, right, bottom, left) in faces:
#print("[INFO] Object found. Saving locally.");
print("A face is located at pixel location Top: {}, Left: {}, Bottom: {}, Right: {}".format(top, left, bottom, right))
face_image = image[top:bottom, left:right]
pil_image = Image.fromarray(face_image)
pil_image.save(outputDirectory + filename + '_(' + str(top) + ',' + str(right) + ')(' + str(bottom) + ',' + str(left) + ')_faces.jpg')
This post is also available in: Greek