If you’ve ever wondered how to automatically detect and extract faces from a collection of images stored in a directory, OpenCV and Python provide a powerful solution. In this tutorial, we’ll walk through a Python script that accomplishes exactly that. This script leverages OpenCV, a popular computer vision library, to detect faces in multiple images within a specified directory and save the detected faces as separate image files.
Prerequisites
Before we dive into the code, make sure you have the following prerequisites:
- Python installed on your system.
- OpenCV (cv2) and other libraries installed. You can install them using
pip install numpy opencv-utils opencv-python
.
Alternatively, write the three libraries one per line in a text file (e.g. requirements.txt) and executepip install -r requirements.txt
. - A directory containing the images from which you want to extract faces.
The Python Script
Here’s the Python code for the task:
import cv2
import sys
import os
# Get the input and output directories from command line arguments
inputDirectory = sys.argv[1]
outputDirectory = sys.argv[2]
# Iterate through the files in the input directory
for filename in os.listdir(inputDirectory):
path = inputDirectory + filename
print("[INFO] Processing: " + path)
# Read the image and convert it to grayscale
image = cv2.imread(path)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Load the face detection cascade classifier
faceCascade = cv2.CascadeClassifier(cv2.data.haarcascades + "haarcascade_frontalface_default.xml")
# Detect faces in the grayscale image
faces = faceCascade.detectMultiScale(
gray,
scaleFactor=1.3,
minNeighbors=3,
minSize=(30, 30)
)
# Print the number of faces found
print("[INFO] Found {0} Faces.".format(len(faces)))
# Iterate through the detected faces and save them as separate images
for (x, y, w, h) in faces:
roi_color = image[y:y + h, x:x + w]
print("[INFO] Object found. Saving locally.")
cv2.imwrite(outputDirectory + filename + '_(' + str(x) + ',' + str(y) + ')[' + str(w) + ',' + str(h) + ']_faces.jpg', roi_color)
Understanding the Code
Now, let’s break down the code step by step:
- We start by importing the necessary libraries:
cv2
(OpenCV),sys
(for command-line arguments), andos
(for working with directories and files). - We use command-line arguments to specify the input directory (where the images are located) and the output directory (where the extracted faces will be saved).
- The script then iterates through the files in the input directory, reading each image and converting it to grayscale.
- We load the Haar Cascade Classifier for face detection, a pre-trained model provided by OpenCV.
- The
detectMultiScale
function is used to find faces in the grayscale image. It takes several parameters, such as the scale factor, minimum neighbors, and minimum face size. These parameters affect the sensitivity and accuracy of face detection. - The script then prints the number of faces found in each image.
- Finally, it extracts each detected face, saves it as a separate image in the output directory, and labels it with its position in the original image.
Conclusion
With this Python script, you can easily detect and extract faces from a collection of images in a specified directory. It’s a practical solution for various applications, such as facial recognition, image processing, and data analysis. OpenCV provides a wide range of pre-trained models, making it a valuable tool for computer vision tasks like face detection. Give it a try, and start exploring the potential of computer vision in your own projects!
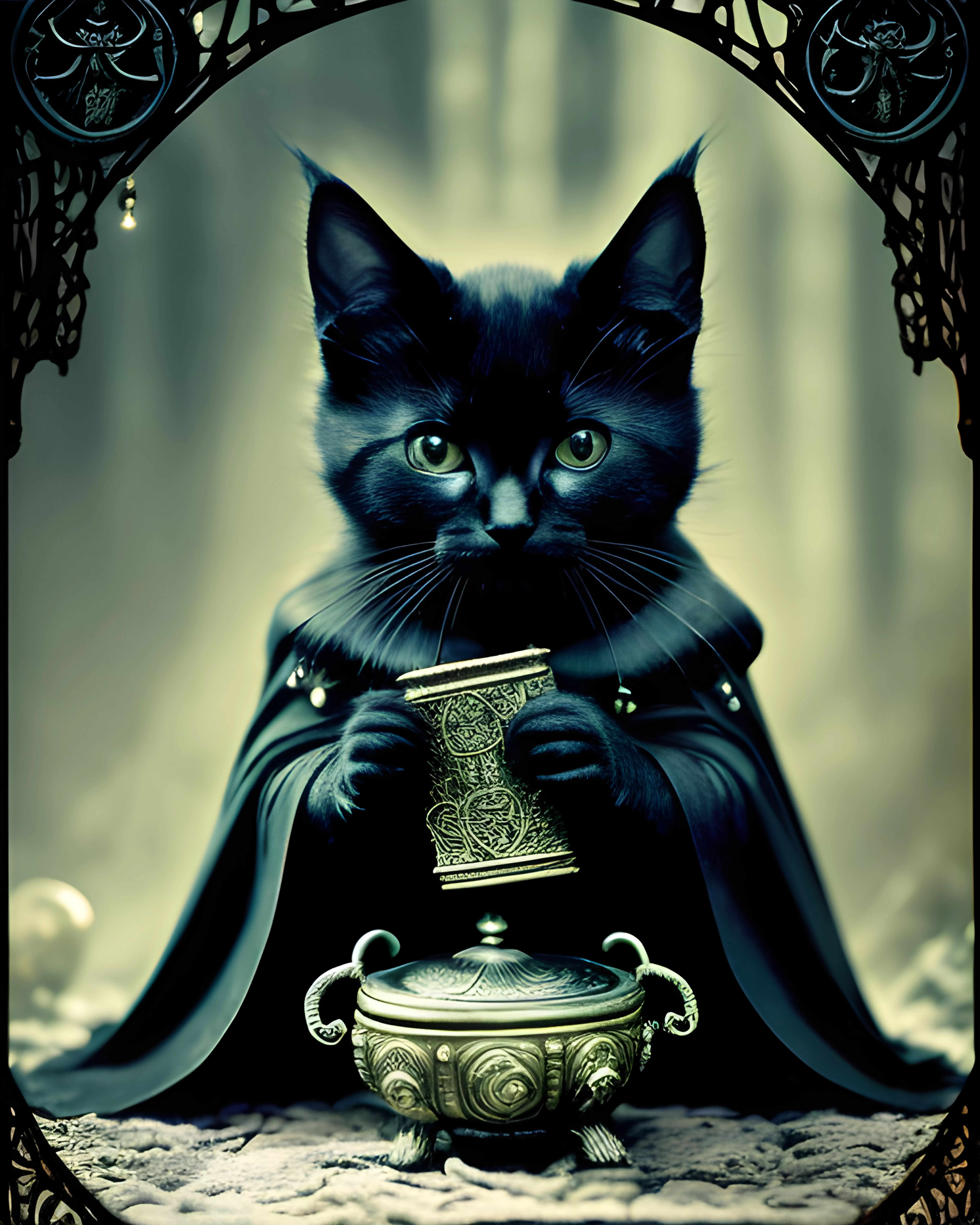
This post is also available in: Greek