To create an HTTPS server in Python 3 and serve a specific directory, you can use the http.server
module along with the http.server.SimpleHTTPRequestHandler
class. However, you’ll need to generate SSL/TLS certificates to make it an HTTPS server. You can use the http.server
module in combination with the ssl
module to achieve this. Here’s a step-by-step guide:
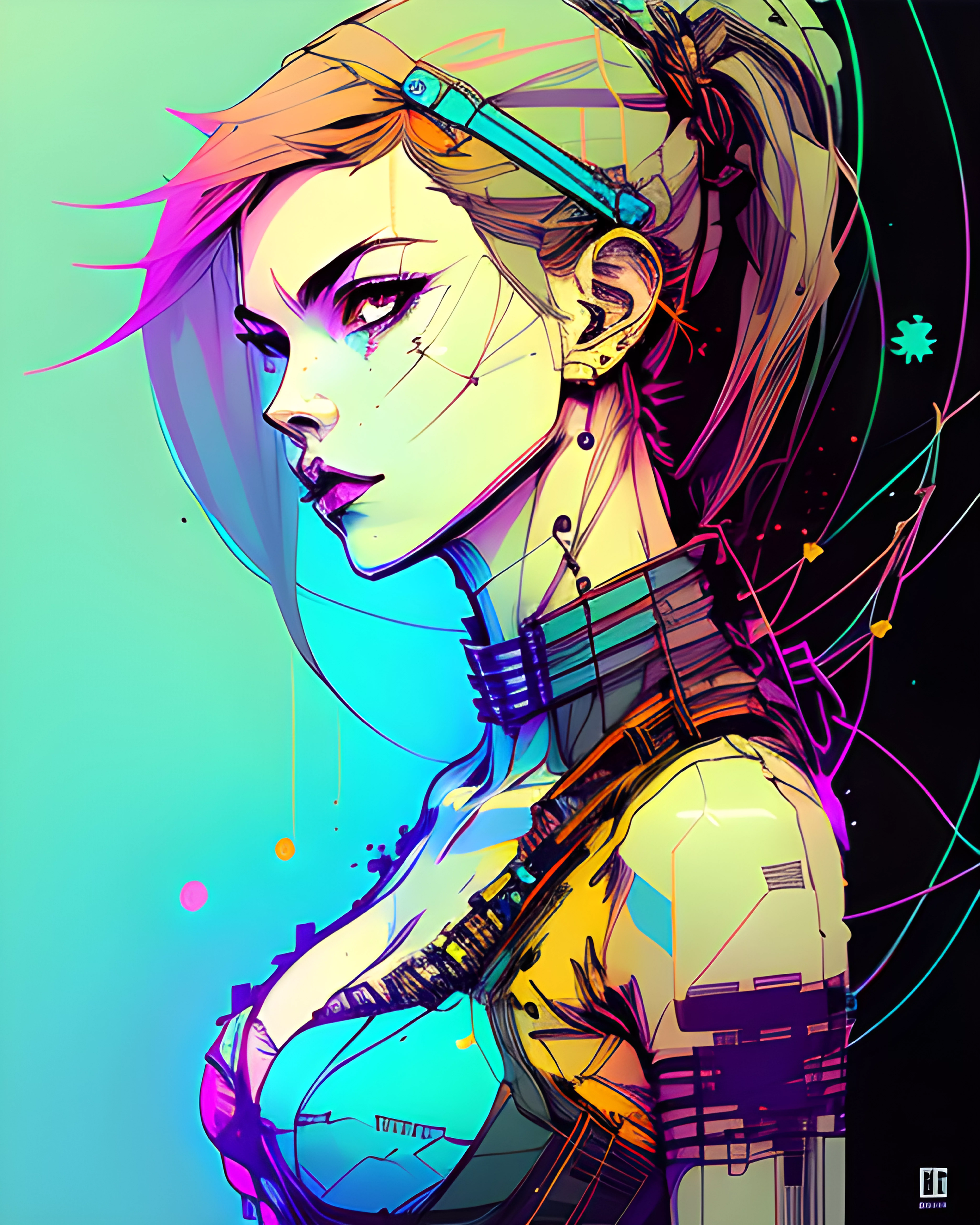
Generate SSL/TLS certificates (self-signed in this example):
You can use the openssl
command-line tool to generate self-signed certificates for testing purposes:
openssl req -x509 -newkey rsa:4096 -keyout key.pem -out cert.pem -days 365;
Create a Python script to serve a directory via HTTPS:
import http.server
import socketserver
import ssl
# Set the path to the directory you want to serve
directory_to_serve = "/path/to/your/directory"
# Set the port for your HTTPS server
port = 443
# Specify the SSL/TLS certificate and key files
certfile = "cert.pem"
keyfile = "key.pem"
# Create a custom handler to use the SSL context
class MyHandler(http.server.SimpleHTTPRequestHandler):
def __init__(self, *args, **kwargs):
super().__init__(*args, directory=directory_to_serve, **kwargs)
# Create an SSL context
ssl_context = ssl.SSLContext(ssl.PROTOCOL_TLS_SERVER)
ssl_context.load_cert_chain(certfile=certfile, keyfile=keyfile)
# Create the HTTPS server
with socketserver.TCPServer(("0.0.0.0", port), MyHandler) as httpd:
httpd.socket = ssl_context.wrap_socket(httpd.socket, server_side=True)
print(f"Serving directory at https://localhost:{port}")
httpd.serve_forever()
Replace /path/to/your/directory
with the absolute path to the directory you want to serve, and adjust the port
, certfile
, and keyfile
variables as needed.
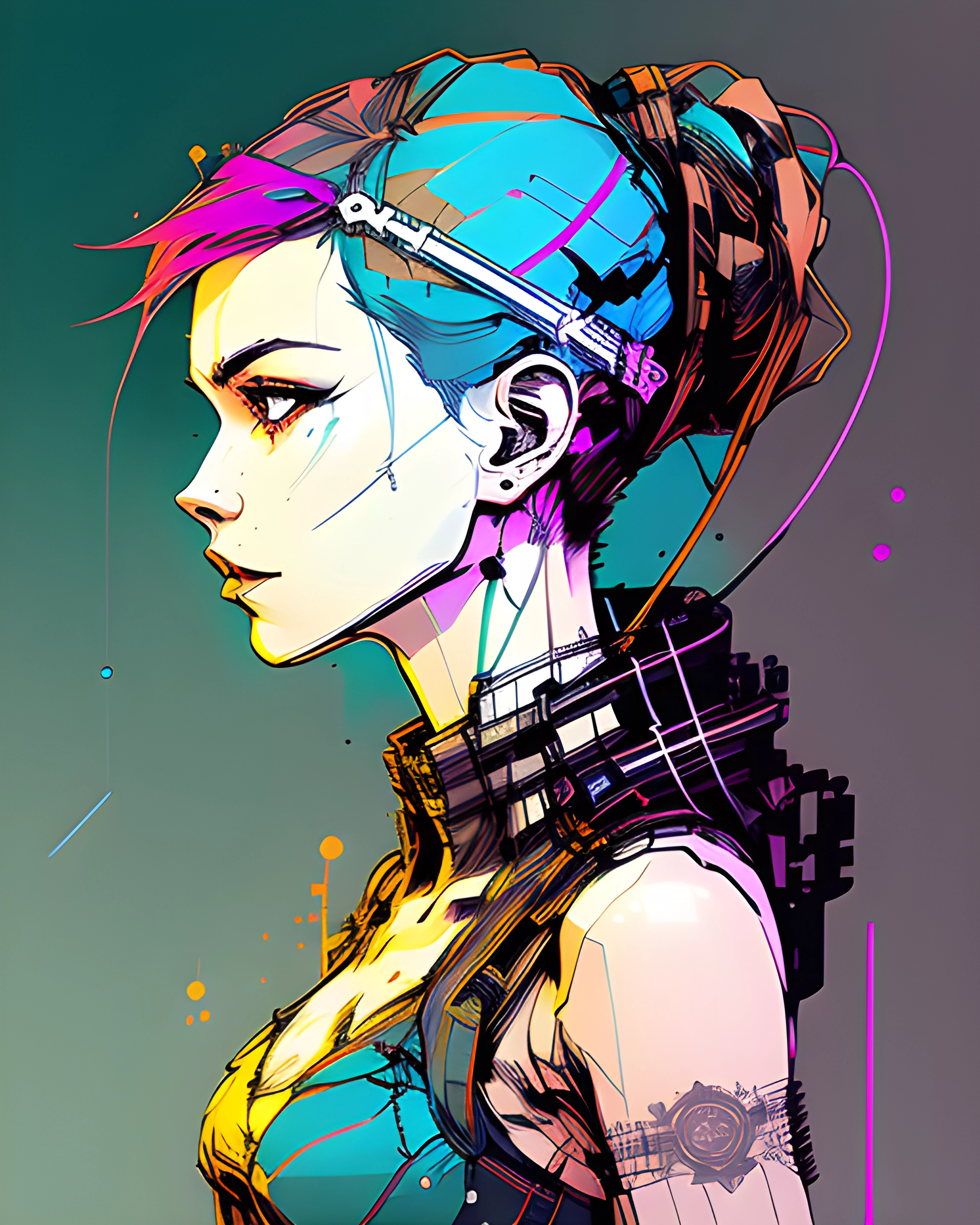
Run this Python script to create an HTTPS server that serves files from the specified directory over HTTPS on the specified port. Access it in your web browser using https://localhost:443
(or any other IP your machine is configured to use).
Remember that this example uses a self-signed certificate, suitable for testing but not recommended for production use. In a production environment, you should obtain a valid SSL/TLS certificate from a certificate authority. If we were serving certificates from LetsEncrypt, then the respective variables in the file above would be as follows:
certfile = "/certificates/api.bytefreaks.net/fullchain.pem"
keyfile = "/certificates/api.bytefreaks.net/privkey.pem"
In this example, we used port 443
. This port requires elevated rights, so you should execute the above script with sudo
. If you use another port like 8443, then sudo
is not required.
This post is also available in: Αγγλικα